How to fix PHP Curl HTTPS Certificate Authority issues on Windows
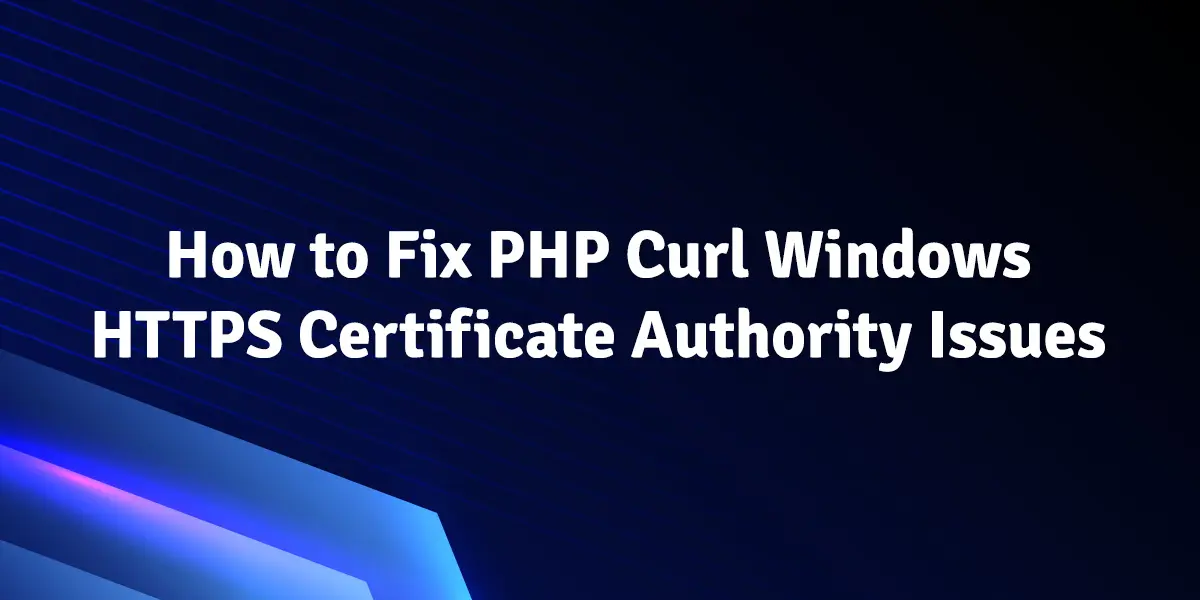
A successful HTTPS request involves the HTTP client validating the server-provided TLS certificate against a list of known and trusted root certificates. The PHP Curl extension is not different; the Curl extension uses libcurl
to make the HTTPS request, and libcurl
, which in turn uses a TLS library such as OpenSSL to validate the request.
The Curl extension requires a valid file containing all of the trusted root certificates to complete the HTTPS validation, and PHP exposes this as a directive in the php.ini
file.
On Linux, BSD, and macOS, libcurl can default to the system root certificates, but this is not possible on Windows because Windows does not come with a single file containing all of the system root certificates.
This article discusses two possible approaches to successfully make HTTPS requests on with the Curl extension, and what not to do that can leave HTTPS requests insecure.
Why it fails
$ch = curl_init('https://php.watch');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_exec($ch); // false
curl_error($ch);
// SSL certificate problem: unable to get local issuer certificate
If the curl_exec
calls fail with a false
response, and if curl_error
indicates an SSL certificate problem: unable to get local issuer certificate
error, this means Curl was not provided a file containing root certificates, or it was unable to discover it.
This error is uncommon on Linux, BSD, and macOS systems, but quite common on Windows because there is no designated file to obtain the root certificates, and the PHP does not ship a root certificate list on its own.
The solution is to provide a file containing up to date root certificates, or ideally, let Curl parse the native root store that the underlying operating system provides.
Use Native Certificate Authorities
On Curl 7.71 and later, it is possible to set a Curl option to request Curl to use the native (system) root certificates. This works even on Windows, where Curl parses system root certificates and uses them.
When CURLOPT_SSL_OPTIONS
option is set to CURLSSLOPT_NATIVE_CA
or a bitmask containing those bits, Curl attempts to use the native root certificate store, subject to the capabilities and versions of the underlying TLS library.
This is the recommended fix, if the Curl extension is built with Curl 7.71
or later and PHP 8.2 and later.
$ch = curl_init('https://php.watch');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
+ curl_setopt($ch, CURLOPT_SSL_OPTIONS, CURLSSLOPT_NATIVE_CA);
curl_exec($ch);
Note the snippet above does not check the Curl version and the PHP version, and assumes both PHP and Curl version requirements are met. The following is an example showing conditionally adding the Curl option:
$ch = curl_init('https://php.watch');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
if (defined('CURLSSLOPT_NATIVE_CA')
&& version_compare(curl_version()['version'], '7.71', '>=')) {
curl_setopt($ch, CURLOPT_SSL_OPTIONS, CURLSSLOPT_NATIVE_CA);
}
curl_exec($ch);
Download and maintain a cacert.pem
file
For applications running on PHP versions older than 8.2 (where CURLSSLOPT_NATIVE_CA
constant is unavailable), or when the Curl version is older than 7.71, the recommended alternative solution is to download a Curl-compatible root certificate file, and configure PHP or the Curl request to use it.
The Curl project maintains an up to date list of certificates. See CA Certificates extracted from Mozilla.
- Download the
cacert.pem
file - Move the file to a directory accessible by PHP and the web server. For example, to
C:/php/cacert.pem
. - Edit the
php.ini
file and modify thecurl.cainfo
entry to point to the absolute path to thecacert.pem
file.[curl] ; A default value for the CURLOPT_CAINFO option. This is required to be an ; absolute path. -;curl.cainfo = +curl.cainfo = "C:/php/cacert.pem"
- Optionally, restart the Web server (such as Apache) to reload the INI file.
The downside of this approach is that the cacert.pem
file must be updated routinely. The cacert.pem
file provided by Curl project, for example, is extracted from the root store maintained by Mozilla. On average, this list and the file get updated 4-5 times a year. To ensure compatibility with the latest root certificates list, make sure to update the local copy of this file regularly.
If it is not possible to modify the INI file, specify the absolute path to the cacert.pem
file within a Curl request as well:
$ch = curl_init('https://php.watch');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
+ curl_setopt($ch, CURLOPT_CAINFO, 'C:/php/cacert.pem');
curl_exec($ch);
On PHP 8.2+ with Curl 7.77, it is possible to a string containing the cacert.pem
contents with the CURLOPT_CAINFO_BLOB
option.
Do NOT Disable Certificate Validation
A common piece of wrong advice found on the Internet forums and articles is to disable the certificate validating. This is a security issue because without certificate validation, Curl will happily accept any TLS certificate, including potentially intercepted or modified contents.
The following example shows such often suggested solution, which is insecure and not recommended.
$ch = curl_init('https://php.watch');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Disables peer certificate validation.
// DO NOT DO THIS!!!
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, 0);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, 0);
curl_exec($ch);